Nullish Coalescing and when you should use it
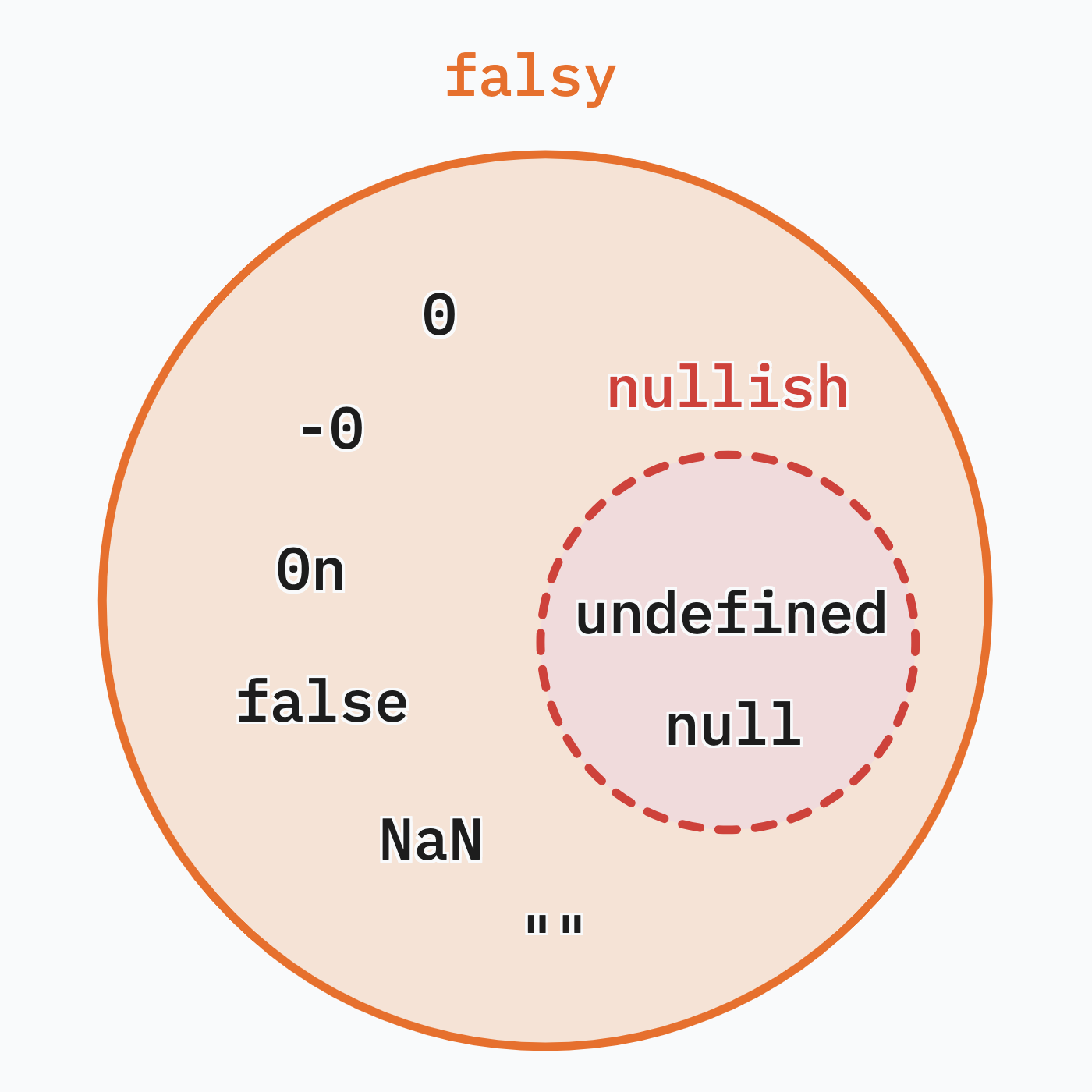
The ??
operator is identical to ||
when the left side is null
, undefined
, or truthy
.
They differ when the left side is falsy
but not null
or undefined
.
In this case:
??
returns the left side.
||
will returns the right side.
In practical terms, ??
returns values with an operable type (string
, number
, bigint
, boolean
, etc.) even when their values are falsy.
falsy
contains nullish
falsy
is a superset of nullish
.
It’s a square, rectangle, paralellagram situation.
Examples
Number
console.log(42 || 'default') // 42console.log(42 ?? 'default') // 42console.log(0 || 'default') // "default"console.log(0 ?? 'default') // 0
String
console.log('juniper' || 'default') // "juniper"console.log('juniper' ?? 'default') // "juniper"console.log('' || 'default') // "default"console.log('' ?? 'default') // ""
Boolean
console.log(true || 'default') // trueconsole.log(true ?? 'default') // trueconsole.log(false || 'default') // "default"console.log(false ?? 'default') // false
Nullish
console.log(undefined || 'default') // "default"console.log(undefined ?? 'default') // "default"console.log(null || 'default') // "default"console.log(null ?? 'default') // "default"