Intersection Observer
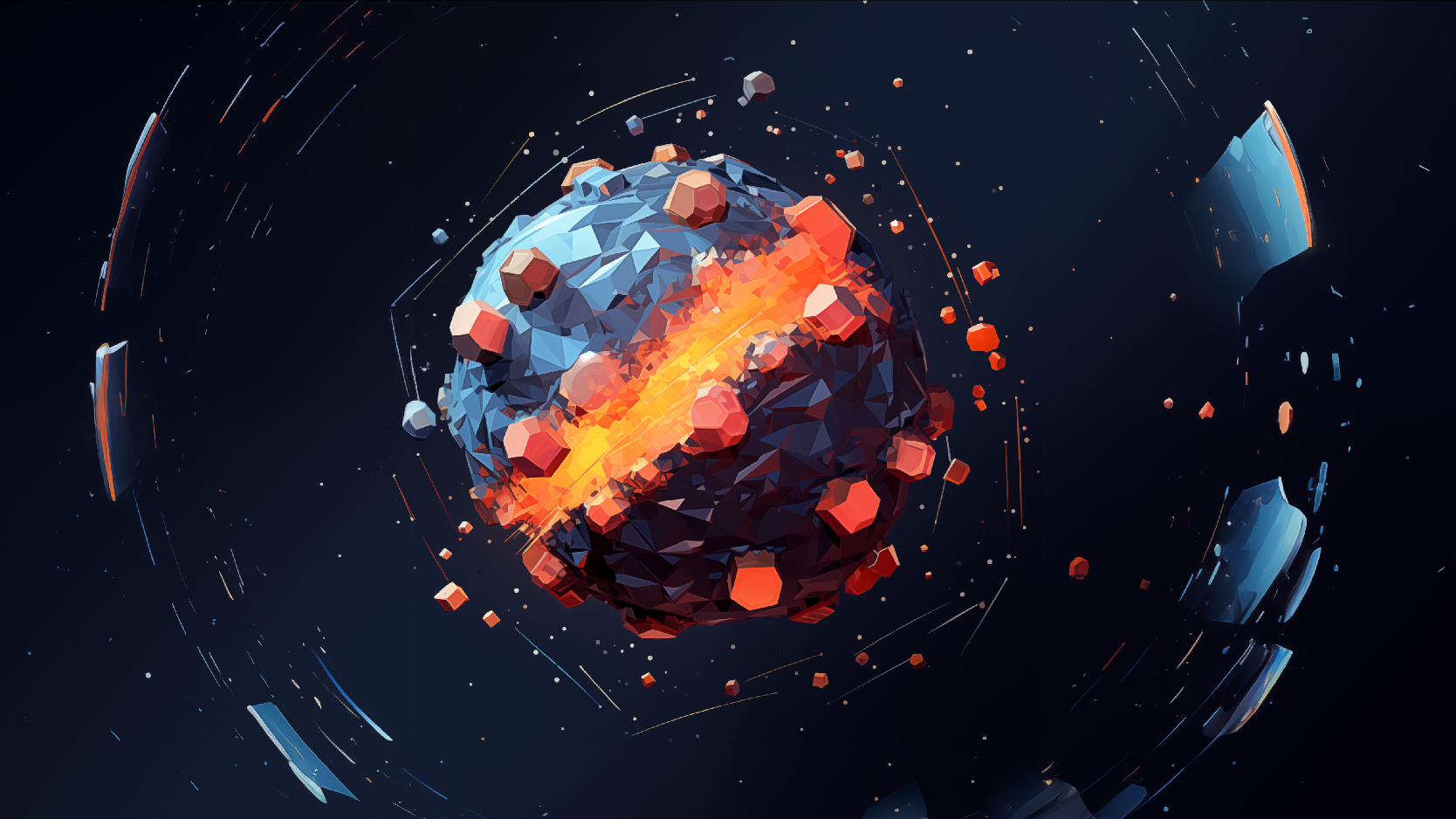
🌱 This post is in the growth phase. It may still be useful as it grows up.
Contents
- Minimum usage
- Instantiate Intersection Observer with options
- Root option
- Threshold option
- Margin option
- IntersectionObserverEntry object
- .observe() method
- .disconnent()
- .takeRecords()
- .unobserve(target)
- Common uses for IntersectionObserver
- When not to use Intersection Observers
- Posts asking for help
- Support
- Cool demo
- Popular videos
Minimum usage
new IntersectionObserver(calledOnIntersection).observe( childElement)
Instantiate Intersection Observer with options
new IntersectionObserver(calledOnIntersection, {})
Root option
root
sets the containing elements. Its default is the top-level viewport.
new IntersectionObserver(calledOnIntersection, { root: document.querySelector('#target_scroll_area'),})
Threshold option
threshold
sets the amount of intersection the observed child must have with the root to trigger the callback. Its default is 0
(any intersection) and can be any decimal between 0
and 1
.
new IntersectionObserver(calledOnIntersection, { root: document.querySelector('#target_scroll_area'), threshold: 0.5, // 50% intersection to be "in view"})
Margin option
margin
accepts any CSS margin value (including negative values). Its default is 0
.
new IntersectionObserver(calledOnIntersection, { root: document.querySelector('#target_scroll_area'), threshold: 0.5, rootMargin: '-50px 0', // 50px of off-screen margin, only on y-axis.})
IntersectionObserverEntry object
Callbacks receive an array of IntersectionObserverEntry objects.
let myIOCallback = (entries, observer) => { entries.forEach((entry) => { // entry: { // boundingClientRect // intersectionRatio // intersectionRect // isIntersecting // rootBounds // target // time // } })}
See these references for details on IntersectionObserverEntry:
.observe() method
Provides an IntersectionObserver instance with target elements to observe.
myIntersectionObserver.observe(target)
Observed multiple elements for intersections:
const descendents = document.querySelectorAll( '.some-descendent')
myIntersectionObserver.observe(targetdescendents)
IntersectionObserver observe() method reference.
.disconnent()
Stops the IntersectionObserver instance from observing any targets.
myIntersectionObserver.disconnect()
IntersectionObserver disconnect() method reference.
.takeRecords()
Query an IntersectionObserver instance for all observed targets. Returns an array of IntersectionObserverEntry objects.
myIntersectionObserver.takeRecords()
IntersectionObserver takeRecords() method reference.
.unobserve(target)
Remove observed target (or targets) from an IntersectionObserver instance.
myIntersectionObserver.unobserve(target)
IntersectionObserver takeRecords() method reference.
Common uses for IntersectionObserver
- “Read Time” and “amount read” indicators.
- Scrolly-telling animation triggers.
- Lazy-loading assets and scripts.
- Prefer more modern tools lazy and script’s
defer async
.
- Prefer more modern tools lazy and script’s
- Closing out-of-view menus, tooltips, and overlays.
- Ad interaction tracking.
When not to use Intersection Observers
Good counterpoints from Ori Livni.
Posts asking for help
Support
Strong: https://caniuse.com/intersectionobserver
Cool demo
Scroll-snap + sticky header
Scrolly-telling
There and back effect
Page navigation highlighting
CodePen collection by Ahmad Shadee
Popular videos
Top YouTube results for "intersection observer"
in incognito.